Welcome to the first post of the TFS Blog Series!
Greg Martin, my friend and
Slalom Consulting collegue, and I will be doing a series of posts about Visual Studio Team System, focused around Team Foundation Server. We will cover a wide range of topics, and hope to give you an in depth look at some of the key features. This series will mostly be from a developer point of view. The majority of the content of the series will apply across both the 2005 and 2008 versions of Team System.
This first post will be an overview. I hope to explain a bit about the what and the why with TFS. The where, when, and how are probably mixed in there somewhere as well.
What is Team System?Visual Studio Team System is an integrated Application Life-cycle Management (ALM) solution. There are several products designed for individuals serving different roles on a project, from Architects to Developers to Testers.
Team System centers around
Team Foundation Server (TFS). TFS is the collaboration server, the "hub" of the system, and integrates all of the supporting products. It stores information about a project and provides project-related services, both figuratively and literally. The services that TFS provides for a project include:
- Work Item Tracking
- Source/Version Control
- Reporting (via SQL Server Reporting Services)
- Build Services (via Team Build)
- Project Portal (via SharePoint)
So what are those other products that make up Team System besides Team Foundation Server? They are the different Team Editions of Visual Studio:
It is important to note that you DO NOT need one of these Team Edtions to use TFS. The regular Visual Studio versions (Standard and Professional) can be used. However, you will miss out on some of the features in the specific editions designed for Team System.
Here is a nice picture provided by Microsoft that visually depicts Team System:
Why should you use it?To me, here are some of the key reasons for why you would want to use TFS on a project.
- Promoting Best Practices
Using TFS promotes you to follow best practices, plain and simple. Because you are provided with just about all the pieces you need to full manage a team project, you would have to make a conscience choice to NOT use features, and not follow best practices. It is entirely opposite when you are not using a product like TFS. Then, you have to make the effort to incorporate best practices into your project. With TFS, its all right there for you to use. And you will.
- Providing One Common Tool Suite
Having a common tool suite is very nice. I personally love not having to leave the Visual Studio IDE to check/modify work items, find documents, view reports, use source control, and run builds. In the past, think about all of the different third party solutions that would come into play. I am sure you probably have worked on a project with VSS or Perforce, NANT, Cruise Control, NUnit, some custom deployment solution, and some sort of Work Item tracking tool. Throwing out all of those in exchange for just ONE product is great. Less time spent getting all of these to play together, and more time spent on the actual delivery of the project.
- What You Get Out of the Box
I love to do a presentation where I show what I get with TFS when starting a new project. I say.... "Lets see what I get in 10 minutes of work". After the 10 minutes I have a new project created, complete with different security groups set up. I have initial Iteration 0 work items assigned to project team members in the Work Item Tracking system. I have some initial documents uploaded into the SharePoint portal for our project. I have reports showing the upcoming work, even displaying in that portal. I have source control set up and my initial code solution imported. And I have an automated, continuous integration build established for that code solution, with the first build complete. IN TEN MINUTES!!! Granted I am talking very fast and I know my way around. But you can see just how much you have at your disposal when starting a new project. No time is wasted getting all of these pieces set up individually.
So on what type of a project would you use TFS? To me, it is for everyone on any software development project, regardless of project size or team size. I have worked on software projects by myself, and I still use TFS. It's great for giving me a source control and a place to plan out and prioritize my work. And if my team of one were to grow, even to two, the project would be all ready for a collaborative work effort. In teams of larger size, it is a no-brainer.
What do I need to get started?The first thing you will need is to identify a Team Foundation Server instance to use. Your company may already have an instance ready for you to use. If you are going to be responsible for installing an instance yourself, check out some
TFS server planning. If you don't have an instace at your disposal, and you don't want to install/maintain one, you aren't totally out of luck. There are TFS hosting providers starting to pop up as well. Check out
TeamDevCentral.
The next step is to install Team Explorer (
2005 version 2008 version ). Team Explorer will allow you to manage the projects and project components of a TFS instance from within Visual Studio. You may be surpised to know that you do not need a specific Team Edition of Visual Studio to install Team Explorer and use TFS. You can actually install Team Explorer without having Visual Studio installed at all. Team Explorer will install the shell itself. If your TFS instance does not have TFS Web Access configured, Team Explorer will be the primary mode of interaction with your team project.
Here is a example of what the Team Explorer window looks like in the IDE:
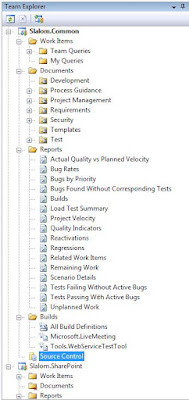
You can see the different areas of Work Items, Documents, Reports, Builds, and Source Control are all nicely viewable in the Visual Studio IDE. One of the nicest things about TFS is its integration within the IDE.
SummaryIf you aren't using TFS, check it out. That's it. I hope you will continue to follow this blog series. There will be some great posts on some specific topics in the near future.
Here are some additonal TFS / Team System resources: